Jest Mocks—Unit Testing for EdgeWorkers
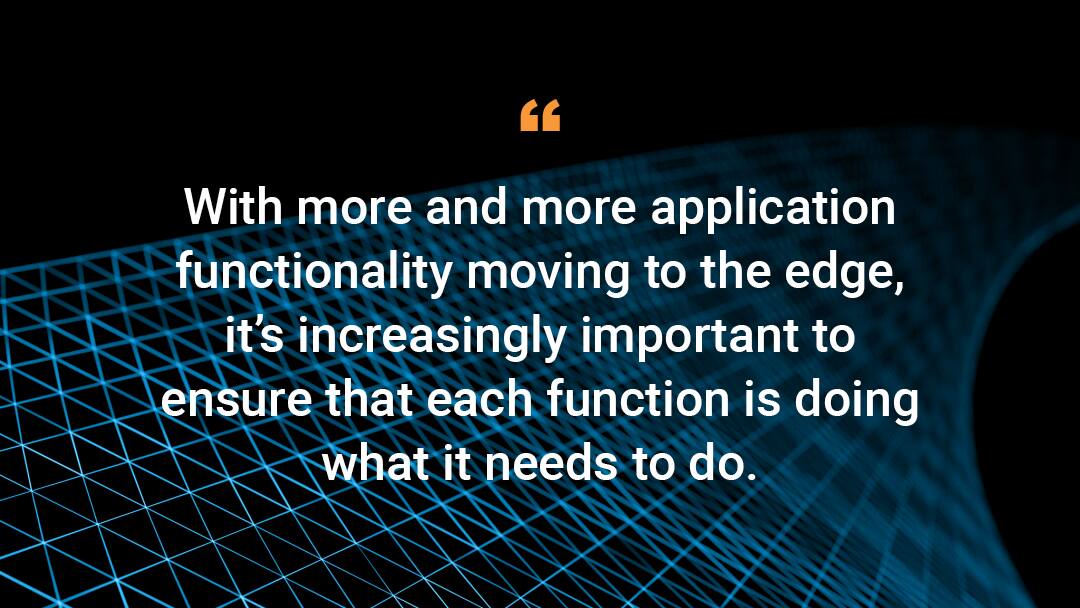
Please note: The steps in this tutorial may change over time. Check our GitHub for the most up-to-date instructions.
In case you haven’t already been working with EdgeWorkers, it allows you to run JavaScript code across more than 4,200 locations for proximity to users and fast application response times.
With more and more application functionality moving to the edge, it’s increasingly important to ensure that each function is doing what it needs to do. A unit test runs code over each segment of your program, checking the input and output. These tests allow developers to check individual areas of a program to see where (and why) errors are occurring. Several frameworks allow you to easily run your tests, including popular frameworks like Jest, Mocha, Jasmine, and Cypress.
With EdgeWorkers, developers can run mock tests at the edge to:
Determine that code is syntactically valid and runs without any issues before it’s published on Akamai
Validate if their business logic is working properly
Test changes behind a company’s secure firewall (without dependency on a remote system)
Be assured that their business logic works, regardless of their code or Akamai’s JavaScript version changes
In this blog, we’ll talk about how you can set up a suite of unit tests with Jest to make sure that your business logic is working properly before deploying changes and to reduce the risk of regressions.
A little background
Jest requires no configuration and is one of the easiest frameworks to learn. Equivalent packages are not available in npm or node, so we created Jest mock examples that allow you to test JavaScript against the EdgeWorkers API in Node.js. Using these manual mocks allows you to have more precise control of their functions.
Our EdgeWorkers repository provides a set of Jest mocks for the EdgeWorkers API. In the Akamai EdgeWorkers execution environment, there are a set of modules and objects provided.
Note: Tests are executed in Node; while both Node and EdgeWorkers run on top of V8, some features are explicitly disabled for EdgeWorkers, there are execution limits for EdgeWorkers (time and memory), and developers need to be careful not to pull in Node APIs.
Ok, now let’s dive into how you can get started.
EdgeWorkers Structure
The EdgeWorker has the following structure:
src — the location of your main.js and bundle.json. All additional modules should go here.
test — unit tests
Create and run tests
Step 1: Start a new project
To create a new project, we’ll execute the command below:
npm init
Step 2: Install Node modules
Here we are going to cover getting the node modules needed to be installed, as well as config file setup.
The mocks for this project are published as the node module edgeworkers-jest-mocks. To install the module, you’ll run the following command:
npm install --save-dev edgeworkers-jest-mocks
Step 3: Set up package.json
Make sure you have the following configured in the package.json file:
Set the test script to Jest
"scripts": {
"test": "jest"
},
Configure Jest
We’ll want to configure Jest first so that the EdgeWorkers API mocks are easier to import.
"jest": {
"testEnvironment": "node",
"transformIgnorePatterns": [
"node_modules/(?!edgeworkers-jest-mocks/__mocks__)"
],
"moduleDirectories": [
"node_modules",
"node_modules/edgeworkers-jest-mocks/__mocks__"
]
}
Step 4: Set up babel.config.json
Babel is included as a dependency to fill in for the newer version of ECMAScript used by Akamai EdgeWorkers. To configure this correctly, we’ll just add the following as a babel.config.json file.
Note: You’ll need to create a JSON file and name it babel.config.json if it doesn’t already exist.
{
"presets": ["@babel/preset-env"],
"plugins": [
["@babel/transform-runtime"]
]
}
Step 5: Write a test
After importing an EdgeWorker or its functions from the main.js file, you can write any kind of tests you need. Tests written against EdgeWorker event handlers require creating a Request or Response mock and then calling the event handler function with that mock.
Here is a quick example of a test written in Jest against an EdgeWorker found in src/main.js:
import * as edgeworker from "../src/main.js";
import Request from 'request';
describe('Simple Example', () => {
beforeEach(() => {
jest.clearAllMocks();
});
test("functional test against onClientRequest", async () => {
let requestMock = new Request();
edgeworker.onClientRequest(requestMock);
expect(requestMock.respondWith).toHaveBeenCalledTimes(1);
expect(requestMock.respondWith).toHaveBeenCalledWith(200, {}, "<html><body><h1>Test Page</h1></body></html>");
});
test("unit test an edgeworker function", async () => {
let result = edgeworker.someFunction(42);
expect(result != null);
});
});
Step 6: Run your tests
Testing is provided via the Jest framework. To run your unit tests, execute the following command from the command line:
npm test
This will run all tests in the test directory following the Jest conventions.
To learn more, look through our resources below. We are continuously growing our list of developer tools and resources to help you simplify the way you work with Akamai.
More resources
If you experience any issues with these code samples, please raise a GitHub issue or chat with one of our developers on the EdgeWorkers Slack space.