How-To: Building a Virtual Python Environment
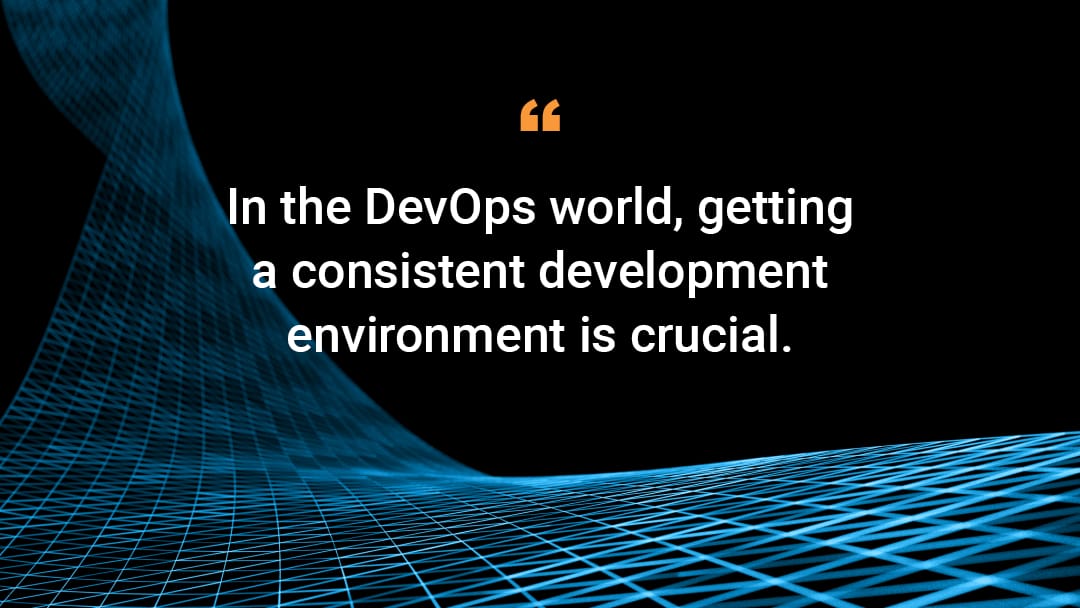
In the DevOps world, getting a consistent development environment is crucial. In this post, I'll show you how to set up a virtual Python environment and install the correct libraries to achieve a consistent development environment.
How to set up a virtual environment
A virtual environment is a tool to keep the dependencies required by different projects in separate places. The virtual environment tool in Python is virtualenv. To work with isolated environments via this tool, let’s examine the basic steps.
Initial setup:
Install the virtualenv tool
Initialize your environment
Install dependencies
Create the requirements.txt to remember the dependencies
Check code and the requirements.txt file into code repository
Developer installs:
Create a virtual environment
Get the latest code
Install dependencies
Start working on code
Now let’s walk through the "initial setup" and "developer installs" stages in a bit more detail.
Initial setup
You or one of the developers on your team can kick start the process by installing the virtualenv tool.
pip install virtualenv
The next step is to initialize the virtual environment on the project folder.
virtualenv myproject
This step will install python, pip, and basic libraries within the project folder, myproject. After version 1.7 of virtualenv, it will use the option –no-site-packages by default. It means that virtualenv will not install the libraries available globally. This will help ensure that the project package contains the bare minimum libraries and the libraries installed for the specific project.
After this, you will need to activate the virtual environment to start working on the project.
source myproject/venv/bin/activate
Once you do this, your prompt will change to show that you are now working in a virtual environment. It should look something like this:
(venv) user@machine $
Now, go ahead and install the libraries that may be required for the project. If all the dependencies can be determined at this stage, it will make it easier to replicate the environment. The dependency list can always be updated as I’ll show later.
pip install <library file>
Once you're done with the installations, create a list of the libraries and their versions.
pip freeze > myproject/requirements.txt
The requirements.txt file will hold the necessary dependencies for your project. In one of my projects, it looks like this:
appdirs==1.4.3
asn1crypto==0.22.0
cffi==1.10.0
cryptography==1.8.1
enum34==1.1.6
idna==2.5
ipaddress==1.0.18
jsontree==0.4.3
ndg-httpsclient==0.4.2
packaging==16.8
pyasn1==0.2.3
pycparser==2.17
pyOpenSSL==17.0.0
pyparsing==2.2.0
requests==2.14.2
six==1.10.0
urllib3==1.21.1
Check this file into your repository along with the project code. If anyone adds functionality which requires a new library, they add it to this requirements.txt file. That way, once other developers pull the update from the source code repository, they'll be aware of the new dependencies and able to install them easily.
Developer install
For each developer, the project setup is now straightforward. To create this virtual environment, first install virtualenv.
pip install virtualenv
Then create a folder and activate a virtual environment.
virtualenv myproject source myproject/venv/bin/activate
After this, check-out the code from the code repository. Assuming it is a git repo, this will just be a git clone command.
git clone
Now navigate to this sub-folder:
(venv) user@machine $ cd myproject/myrepo
Install the dependencies:
pip -r requirements.txt
That’s it. Your developer machine is now all set for coding. Happy Python-ing!
Learn more
This page on The Hitchhiker's Guide to Python is an excellent reference for anything virtualenv.