Say Goodbye to Monolithic EdgeWorkers: Introducing Flexible Composition (Part 1)
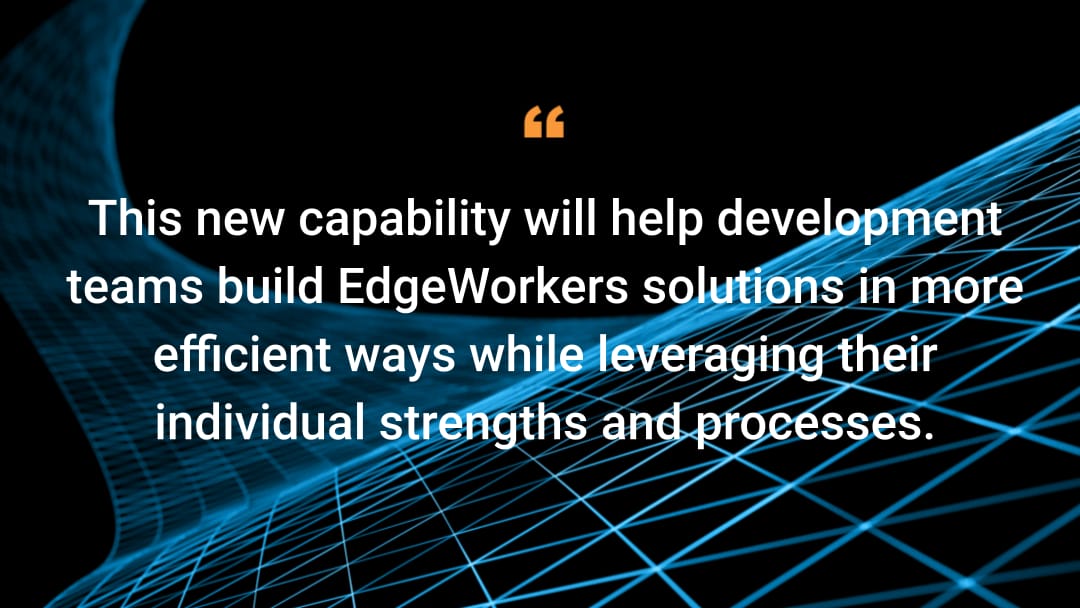
Soon, it will be easier for companies with multiple development teams to build EdgeWorkers solutions together. Until now, only a single EdgeWorker could handle a request, meaning all the logic for managing the request had to be developed, tested, debugged, activated, and monitored together. Although that’s okay for small teams that have a single continuous integration (CI) pipeline, large organizations rarely have that ability.
Many companies have several development teams with separate CI pipelines, use different tools, deliver on different schedules, and may even build in different languages. For more complex development shops, working in a single EdgeWorkers codebase can be cumbersome, inefficient, and error-prone — with a unique learning curve, as well.
A new EdgeWorkers feature
Akamai recently rolled out a functionality called Flexible Composition (currently available in beta) for companies with multiple teams. Using Flexible Composition, developers can write a single parent EdgeWorker that receives a request and calls in child EdgeWorkers as appropriate (Figure 1).
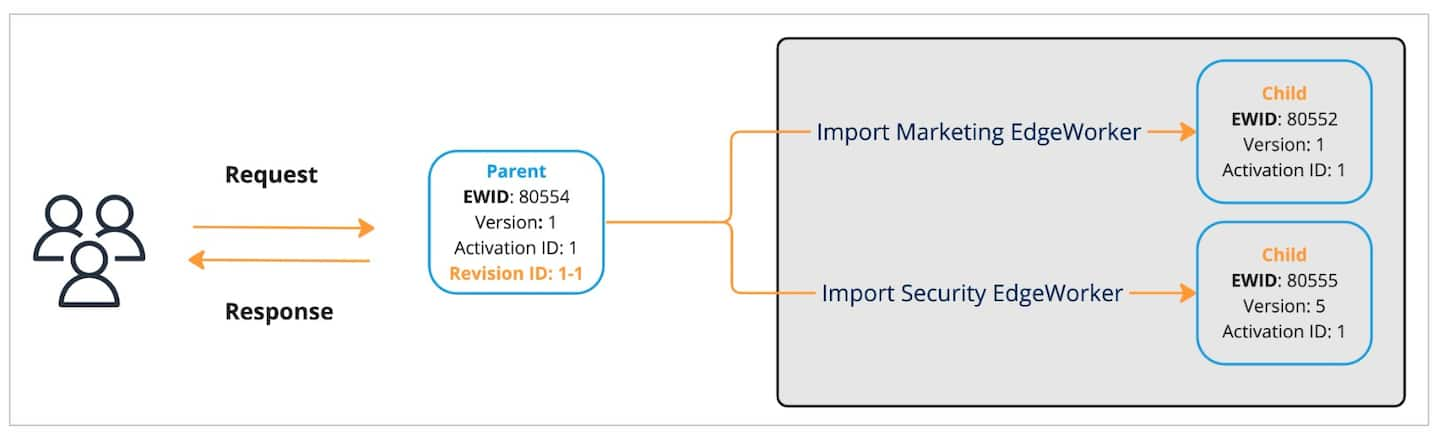
Each team can develop their own EdgeWorker, using their preferred tools and delivering on their desired schedule. When a team activates their EdgeWorker on the network, all parent EdgeWorkers that use it are dynamically reactivated to leverage the new changes. Dynamic reactivation occurs automatically, so EdgeWorkers developers don't need to worry about managing release cycles or coordinating changes.
The benefits of Flexible Composition
This new capability will help development teams build EdgeWorkers solutions in more efficient ways while leveraging their individual strengths and processes. Teams can reap the benefits of:
Independent contribution. Each team can work at its own pace. Developers can activate a new version of their EdgeWorkers function anytime they need to add functionality to the website. They don't need to coordinate with other teams.
Dynamic activation. The parent EdgeWorker automatically receives updates. The owner doesn't need to rebuild, upload, or run a CI pipeline to activate it.
EdgeWorkers as separately imported files. Each child EdgeWorker is represented as a JavaScript module.
- Friction-free code reuse. Child EdgeWorkers can export any type of JavaScript object, including classes and functions.
- A choice of developer tools. Each team in the organization can use their preferred developer tools. If developers want to build their EdgeWorkers in TypeScript, they can do so without having to integrate into a larger build chain.
Flexible Composition in action
Let’s assume the fictional Acme Corp is running a digital commerce store. Acme’s website is complex, with separate teams handling the search engine optimization (SEO), marketing, and security of the same site.
import * as seo from "seo";
import * as marketing from "marketing";
import * as security from "security";
// ...
export async function responseProvider(request) {
let product = marketing.getProduct(request);
if (seo.isCrawler(request)) {
return respondWith(200, seo.indexableOutput(product));
}
let user = security.login(request);
let page = new PageStream();
page.setProduct(product);
marketing.addDealsFor(request, user, page);
return createResponse(200, page.toStream());
}
Using Flexible Composition, each team defines their own EdgeWorker, and a top-level (or “parent”) EdgeWorker pulls the site together (Figure 2).
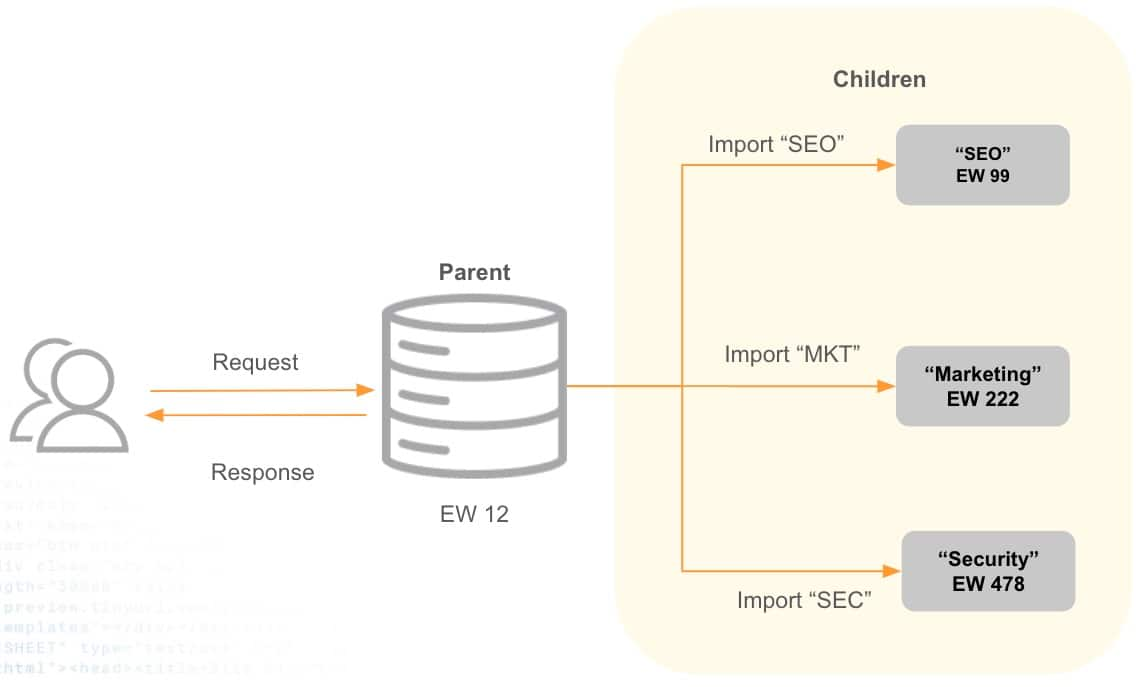
How does it work?
What if the marketing team wants to add some functionality to the site? Perhaps they want to localize an ad campaign to a specific region. How does that work in this new interconnected configuration? The team can just modify the addDealsFor() function in their EdgeWorker with the new logic. After testing the changes, they can activate the new version on the Akamai staging environment.
As soon as the marketing EdgeWorker becomes active, the staging version of the parent EdgeWorker is dynamically reactivated to include the marketing EdgeWorker. That means a request sent to the parent EdgeWorker will use the version of addDealsFor() with the new localization changes.
Leveraging package management at the edge
Essentially, Flexible Composition allows developers to leverage package management at the edge. Like npm, Gradle, and pip, each EdgeWorker declares a set of dependencies in its bundle.json. When the EdgeWorker is loaded, the dependencies are loaded as modules.
The parent EdgeWorker from our Acme example would contain:
dependencies: {
seo: {
edgeWorkerId: 99,
version: "active"
},
marketing: {
edgeWorkerId: 222,
version: "active"
},
security: {
edgeWorkerId: 478,
version: "active"
}
}
Each key is the importable module name, and the value indicates the version of the dependency that should be loaded. That means the EdgeWorker can use import * as seo from "seo" to load the exports from EdgeWorker 99.
Child EdgeWorkers may also contain dependencies. Perhaps Acme has a support team that provides an input sanitizer to prevent cross-site scripting (XSS) attacks. The marketing EdgeWorker handles user reviews, so it uses the XSS module by declaring a dependency in the bundle.json:
dependencies: {
xss: {
edgeWorkerId: 1356,
version: "active"
}
}
When a new version of the XSS module is activated, all the EdgeWorkers that import it are dynamically reactivated, as are the EdgeWorkers that import those. During dynamic reactivation, the parent EdgeWorkers that use the XSS module are updated to include the active version and are redeployed to the Akamai network. That means the support team can fix the bug and then activate in the XSS EdgeWorker, at which point all Acme's EdgeWorkers will be updated to use the fixed version.
With the XSS module from the support team, the dependency hierarchy looks like:
parent EdgeWorker
+ seo (EW 99)
+ marketing (EW 222)
+ xss (EW 1356)
+ security (EW 478)
Summary
By deconstructing EdgeWorkers multiple teams can work together efficiently using existing company processes, which can contribute to a single-edge application solution or split edge code into more easily editable pieces. Dynamic reactivation streamlines the process of getting changes to production, so teams that own a parent EdgeWorker don’t have to monitor and reactivate it every time a child changes.
Stay tuned
Stay tuned for a follow-up blog post with more information about Flexible Composition. This feature was rolled out in early November 2023, and is available now as a beta. If you’d like to participate in the beta program, please contact your Akamai representative.
Additional development on Flexible Composition continues with a focus on security features and the ability to import specific EdgeWorkers versions. Read more about this exciting new feature on our TechDocs site.
EdgeWorkers Flexible Composition TechDocs
Terminology
Create a parent EdgeWorker